2023. 1. 4. 12:00ㆍ공부/Android Studio
이전 포스팅에서 notification을 띄우면서 백그라운드 작업을 진행하는 포어그라운드 서비스에 대해서 공부했었다.
https://growth-coder.tistory.com/48
[Android/Kotlin] Foreground Service 사용 방법
음악을 틀어놓고 웹 서핑을 한다거나 애플리케이션을 설치하면서 문자를 한다거나 이렇게 특정 동작을 수행 도중에 다른 동작도 수행할 일이 있을 수 있다. 위의 예시에서 음악, 앱 설치의 경우
growth-coder.tistory.com
이번에는 notification을 띄우지 않고 백그라운드 작업을 진행해보려 한다.
버튼을 클릭하면 notification을 띄우지 않고 백그라운드에서 1에서 100까지 로그를 출력해보려 한다.
이번에 사용할 Work Manager는 좋지 않은 환경에서 잠시 작업을 멈추었다가 좋은 환경일 때 다시 작업을 진행하도록 할 수 있다.
work manager의 경우 제한 상황을 명확히 정해두는 편이 좋다.
먼저 dependency를 추가해야한다.
build.gradle의 dependencies에 다음 코드를 추가해준다.
implementation 'androidx.work:work-runtime-ktx:2.7.1'
<build.gradle (:app)>
dependencies{
.
.
.
implementation 'androidx.work:work-runtime-ktx:2.7.1'
}
이제 Worker를 상속받은 클래스를 만들 차례이다.
class WorkerPractice(context: Context, workerParameters : WorkerParameters)
: Worker(context,workerParameters){
override fun doWork(): Result {
TODO("Not yet implemented")
}
}
파라미터로 context와 workerParameters를 받아주고 doWork를 오버라이딩 해준다.
class WorkerPractice(context: Context, workerParameters : WorkerParameters)
: Worker(context,workerParameters){
override fun doWork(): Result {
TODO("Not yet implemented")
}
}
이제 doWork 메소드에서 1부터 100까지 로그를 출력하는 코드를 작성하면 된다.
포어그라운드 서비스에서는 delay를 썼었는데 여기에서는 sleep을 써도 된다.
코드를 작성하고 나서는 Result.success()를 반환해준다.
class WorkerPractice(context: Context, workerParameters : WorkerParameters)
: Worker(context,workerParameters){
override fun doWork(): Result {
for(i in 1..100){
Log.d("worker practice", i.toString())
sleep(100)
}
return Result.success()
}
}
이제 activity_main.xml 에서 아이디가 button인 버튼을 만들어준다.
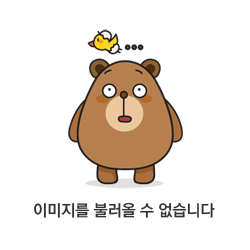
<activity_main.xml>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="실행"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
클릭 이벤트를 만들어준다.
class MainActivity : AppCompatActivity() {
private lateinit var binding: ActivityMainBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
binding.button.setOnClickListener{
}
}
}
setOnClickLister 안에 constraints를 만들어준다.
제한 사항은 네트워크 연결은 상관없고 충전 중일때만 작업을 진행하도록 설정해준다.
val constraints = Constraints.Builder()
.setRequiredNetworkType(NetworkType.NOT_REQUIRED)
.setRequiresCharging(true)
.build()
한 번만 실행할거라면 OneTimeWorkRequest를 주기적으로 실행할 거라면 PeriodicWorkRequest를 사용한다.
한 번만 실행시키는 코드를 작성하려한다.
val oneTimeWorkRequest = OneTimeWorkRequest.Builder(WorkerPractice::class.java)
.setConstraints(constraints)
.build()
WorkManager.getInstance(this).enqueue(oneTimeWorkRequest)
'공부 > Android Studio' 카테고리의 다른 글
[Android/Kotlin] AlarmManager 사용법 (2) | 2023.01.05 |
---|---|
[Android/Kotlin] 리사이클러 뷰 아이템에 애니메이션 (view binding) (0) | 2023.01.03 |
[Android/Kotlin] Foreground Service 사용 방법 (0) | 2022.12.24 |
[Android/Kotlin] MVVM Repository 패턴을 활용하여 데이터베이스의 유저 정보 등록 및 출력 (0) | 2022.12.23 |
[Android Studio] Firebase와 연결하기 (0) | 2022.12.13 |